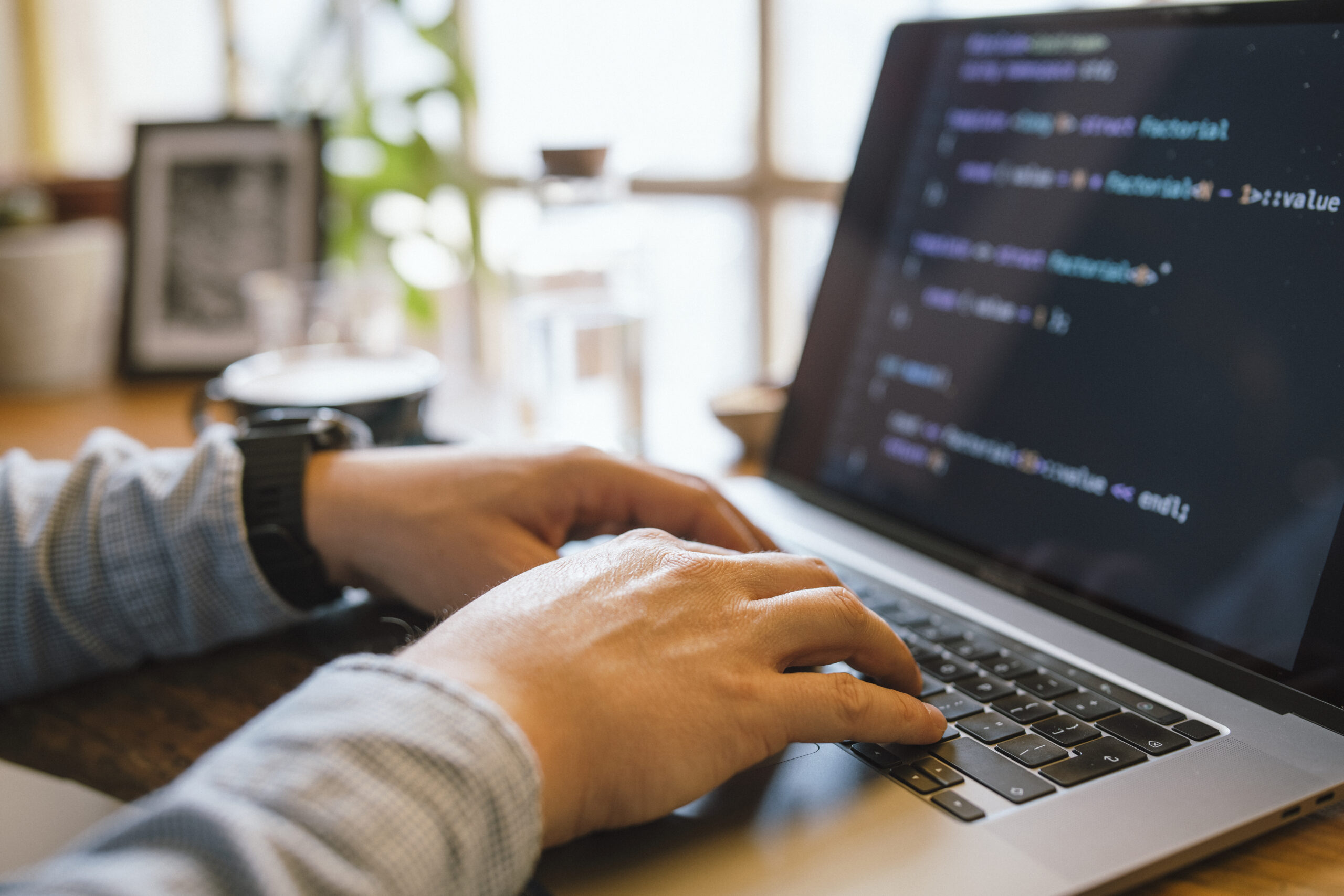
Debugging is Just about the most vital — yet frequently disregarded — competencies in a developer’s toolkit. It isn't nearly fixing damaged code; it’s about comprehending how and why items go Mistaken, and Mastering to Imagine methodically to unravel problems efficiently. Regardless of whether you're a novice or possibly a seasoned developer, sharpening your debugging capabilities can help you save several hours of frustration and significantly enhance your productivity. Listed here are a number of methods to assist developers level up their debugging game by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies builders can elevate their debugging techniques is by mastering the equipment they use on a daily basis. Even though producing code is just one Element of enhancement, knowing how to interact with it successfully during execution is equally crucial. Present day improvement environments come equipped with effective debugging capabilities — but many builders only scratch the floor of what these resources can perform.
Just take, by way of example, an Integrated Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code about the fly. When utilized the right way, they Enable you to observe exactly how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish developers. They allow you to inspect the DOM, check community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, resources, and community tabs can turn annoying UI issues into manageable jobs.
For backend or procedure-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Handle around working procedures and memory administration. Studying these instruments may have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, develop into comfortable with Edition Management units like Git to know code historical past, come across the precise instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments means going past default options and shortcuts — it’s about building an intimate understanding of your growth natural environment to make sure that when issues arise, you’re not lost in the dark. The better you realize your resources, the more time you'll be able to commit fixing the actual difficulty as an alternative to fumbling by the method.
Reproduce the situation
Among the most significant — and infrequently forgotten — techniques in productive debugging is reproducing the problem. Just before jumping to the code or generating guesses, builders need to produce a dependable ecosystem or state of affairs where the bug reliably seems. Without the need of reproducibility, repairing a bug will become a match of possibility, usually leading to squandered time and fragile code alterations.
The first step in reproducing a problem is accumulating as much context as possible. Ask questions like: What steps led to The difficulty? Which setting was it in — growth, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it turns into to isolate the precise situations under which the bug happens.
Once you’ve gathered sufficient information and facts, try and recreate the issue in your neighborhood ecosystem. This could necessarily mean inputting precisely the same data, simulating related user interactions, or mimicking technique states. If the issue appears intermittently, take into consideration creating automatic tests that replicate the sting instances or condition transitions associated. These exams don't just assist expose the situation but additionally reduce regressions Later on.
From time to time, The difficulty could be natural environment-particular — it would materialize only on specific running programs, browsers, or less than particular configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t merely a move — it’s a state of mind. It calls for endurance, observation, in addition to a methodical approach. But when you can persistently recreate the bug, you happen to be by now midway to correcting it. Which has a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and converse far more Plainly with the staff or people. It turns an summary grievance right into a concrete problem — and that’s in which developers thrive.
Go through and Fully grasp the Mistake Messages
Error messages are sometimes the most useful clues a developer has when anything goes Mistaken. As an alternative to viewing them as aggravating interruptions, developers ought to learn to take care of mistake messages as direct communications in the system. They normally inform you just what occurred, where it transpired, and often even why it occurred — if you know how to interpret them.
Get started by looking at the concept carefully As well as in entire. A lot of developers, especially when less than time strain, glance at the very first line and immediately get started building assumptions. But deeper while in the error stack or logs might lie the true root cause. Don’t just duplicate and paste error messages into search engines like yahoo — study and have an understanding of them first.
Split the mistake down into elements. Could it be a syntax error, a runtime exception, or possibly a logic error? Will it issue to a selected file and line range? What module or perform brought on it? These inquiries can guidebook your investigation and point you toward the liable code.
It’s also handy to grasp the terminology from the programming language or framework you’re using. Mistake messages in languages like Python, JavaScript, or Java usually stick to predictable styles, and Understanding to acknowledge these can substantially speed up your debugging system.
Some mistakes are imprecise or generic, and in those situations, it’s crucial to examine the context in which the error transpired. Check relevant log entries, enter values, and recent alterations in the codebase.
Don’t forget about compiler or linter warnings possibly. These typically precede bigger troubles and provide hints about likely bugs.
Finally, error messages are certainly not your enemies—they’re your guides. Learning to interpret them appropriately turns chaos into clarity, encouraging you pinpoint problems more quickly, lessen debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is The most highly effective applications inside a developer’s debugging toolkit. When utilized efficiently, it offers authentic-time insights into how an application behaves, aiding you recognize what’s occurring underneath the hood without having to pause execution or move through the code line by line.
A great logging method begins with being aware of what to log and at what stage. Prevalent logging concentrations include DEBUG, INFO, WARN, ERROR, and FATAL. Use DEBUG for specific diagnostic information in the course of progress, INFO for typical events (like effective begin-ups), WARN for opportunity problems that don’t break the appliance, Mistake for genuine challenges, and Deadly when the system can’t continue on.
Prevent flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure important messages and decelerate your technique. Give attention to key situations, condition modifications, input/output values, and critical final decision factors in your code.
Structure your log messages Obviously and consistently. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace challenges in distributed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Enable you to monitor how variables evolve, what disorders are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Particularly precious in manufacturing environments wherever stepping by means of code isn’t probable.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, wise logging is about stability and clarity. Which has a effectively-believed-out logging method, you could lessen the time it will take to identify issues, get deeper visibility into your apps, and Increase the General maintainability and trustworthiness of one's code.
Imagine Just like a Detective
Debugging is not merely a technical activity—it's a type of investigation. To proficiently identify and resolve bugs, builders must strategy the method similar to a detective solving a mystery. This mentality assists stop working complex problems into manageable sections and adhere to clues logically to uncover the foundation cause.
Commence by collecting proof. Think about the symptoms of the trouble: mistake messages, incorrect output, or general performance problems. The same as a detective surveys against the law scene, gather as much pertinent data as you could with out jumping to conclusions. Use logs, examination cases, and person experiences to piece collectively a clear picture of what’s occurring.
Upcoming, form hypotheses. Talk to yourself: What may very well be creating this behavior? Have any modifications lately been built to your codebase? Has this issue happened before less than similar circumstances? The intention will be to narrow down choices and recognize prospective culprits.
Then, exam your theories systematically. Seek to recreate the situation in a very managed surroundings. In case you suspect a selected function or ingredient, isolate it and confirm if the issue persists. Like a detective conducting interviews, request your code concerns and Permit the effects guide you nearer to the reality.
Pay out close awareness to modest information. Bugs frequently disguise while in the least expected sites—similar to a lacking semicolon, an off-by-just one error, or maybe a race affliction. Be comprehensive and individual, resisting the urge to patch The difficulty with no totally being familiar with it. Momentary fixes might disguise the true trouble, only for it to resurface later on.
Lastly, hold notes on what you experimented with and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and assist Some others comprehend your reasoning.
By imagining similar to a detective, builders can sharpen their analytical skills, technique complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated devices.
Write Exams
Producing checks is among the most effective approaches to transform your debugging skills and General growth performance. Checks not only assist catch bugs early but in addition serve as a security Internet that offers you assurance when making modifications in your codebase. A properly-examined application is much easier to debug mainly because it allows you to pinpoint precisely in which and when a difficulty happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can swiftly reveal no matter whether a certain piece of logic is Functioning as anticipated. Whenever a check fails, you instantly know exactly where to look, significantly reducing some time expended debugging. Device exams are Particularly helpful for catching regression bugs—issues that reappear after Beforehand staying mounted.
Up coming, integrate integration checks and conclusion-to-conclude exams into your workflow. These help make sure several areas of your application do the job alongside one another efficiently. They’re specifically useful for catching bugs that manifest in intricate techniques with multiple parts or providers interacting. If something breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Composing checks also forces you to think critically regarding your code. To test a attribute effectively, you need to be aware of its inputs, anticipated outputs, and edge conditions. This amount of understanding In a natural way prospects to raised code construction and much less bugs.
When debugging an issue, producing a failing check that reproduces the bug is often a powerful initial step. As soon as the examination fails consistently, it is possible to focus on repairing the bug and check out your check move when The difficulty is settled. This tactic ensures that precisely the same bug doesn’t return Down the road.
Briefly, writing exams turns debugging from a disheartening guessing recreation right into a structured and predictable process—encouraging you capture far more bugs, more rapidly and a lot more reliably.
Get Breaks
When debugging a difficult challenge, it’s simple to become immersed in the situation—gazing your screen for hours, striving Option just after solution. But Just about the most underrated debugging instruments is actually stepping absent. Getting breaks allows you reset your mind, decrease frustration, and often see the issue from a new perspective.
When you're as well close to the code for as well extended, cognitive fatigue sets in. You could commence overlooking clear problems or misreading code that you just wrote just hrs previously. On this read more state, your Mind gets considerably less productive at dilemma-fixing. A short walk, a espresso split, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. Quite a few developers report locating the root of a problem when they've taken time and energy to disconnect, allowing their subconscious operate inside the track record.
Breaks also help reduce burnout, Primarily through more time debugging sessions. Sitting down before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps out of the blue recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a five–10 moment break. Use that point to maneuver all over, stretch, or do a thing unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it really in fact causes more quickly and more practical debugging Eventually.
To put it briefly, getting breaks is not really a sign of weak spot—it’s a smart approach. It presents your brain Place to breathe, increases your viewpoint, and can help you avoid the tunnel vision That always blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Master From Every Bug
Just about every bug you encounter is more than just A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or maybe a deep architectural issue, each one can educate you a thing useful when you take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a couple of crucial inquiries when the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device tests, code opinions, or logging? The responses often expose blind places in the workflow or knowledge and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see styles—recurring troubles or widespread blunders—which you can proactively steer clear of.
In team environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. No matter whether it’s through a Slack information, a brief compose-up, or a quick know-how-sharing session, supporting Other individuals avoid the similar concern boosts team performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important aspects of your growth journey. In the end, many of the greatest builders usually are not those who compose excellent code, but those who repeatedly learn from their problems.
In the end, Just about every bug you repair provides a new layer to the talent set. So future time you squash a bug, take a minute to reflect—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Improving upon your debugging expertise usually takes time, follow, and tolerance — however the payoff is large. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.